Day 2 Operators Hackerrank Solution is part of Hackerrank’s 30 days of code, in this problem. Given the meal price (base cost of a meal), tip per cent (the percentage of the meal price being added as a tip), and tax per cent (the percentage of the meal price being added as tax) for a meal, find and print the meal’s total cost.
Note: Be sure to use precise values for your calculations, or you may end up with an incorrectly rounded result!
Table of Contents
Day 2 Operators Hackerrank Solution in C
#include <assert.h>
#include <limits.h>
#include <math.h>
#include <stdbool.h>
#include <stddef.h>
#include <stdint.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
char* readline();
// Complete the solve function below.
void solve(double meal_cost, int tip_percent, int tax_percent)
{
double tip=meal_cost*tip_percent/100;
double tax=meal_cost*tax_percent/100;
int totalCost=(int)round(meal_cost+tip+tax);
printf("%d",totalCost);
}
int main()
{
char* meal_cost_endptr;
char* meal_cost_str = readline();
double meal_cost = strtod(meal_cost_str, &meal_cost_endptr);
if (meal_cost_endptr == meal_cost_str || *meal_cost_endptr != '\0')
{
exit(EXIT_FAILURE);
}
char* tip_percent_endptr;
char* tip_percent_str = readline();
int tip_percent = strtol(tip_percent_str, &tip_percent_endptr, 10);
if (tip_percent_endptr == tip_percent_str || *tip_percent_endptr != '\0')
{
exit(EXIT_FAILURE);
}
char* tax_percent_endptr;
char* tax_percent_str = readline();
int tax_percent = strtol(tax_percent_str, &tax_percent_endptr, 10);
if (tax_percent_endptr == tax_percent_str || *tax_percent_endptr != '\0')
{
exit(EXIT_FAILURE);
}
solve(meal_cost, tip_percent, tax_percent);
return 0;
}
char* readline() {
size_t alloc_length = 1024;
size_t data_length = 0;
char* data = malloc(alloc_length);
while (true) {
char* cursor = data + data_length;
char* line = fgets(cursor, alloc_length - data_length, stdin);
if (!line) { break; }
data_length += strlen(cursor);
if (data_length < alloc_length - 1 || data[data_length - 1] == '\n')
{
break;
}
size_t new_length = alloc_length << 1;
data = realloc(data, new_length);
if (!data) { break; }
alloc_length = new_length;
}
if (data[data_length - 1] == '\n')
{
data[data_length - 1] = '\0';
}
data = realloc(data, data_length);
return data;
}
Solution Day 2 Operators in C++
#include <bits/stdc++.h>
using namespace std;
// Complete the solve function below.
void solve(double meal_cost, int tip_percent, int tax_percent)
{
double tip=meal_cost*tip_percent/100;
double tax=meal_cost*tax_percent/100;
int totalCost=(int)round(meal_cost+tip+tax);
cout<<totalCost;
}
int main()
{
double meal_cost;
cin >> meal_cost;
cin.ignore(numeric_limits<streamsize>::max(), '\n');
int tip_percent;
cin >> tip_percent;
cin.ignore(numeric_limits<streamsize>::max(), '\n');
int tax_percent;
cin >> tax_percent;
cin.ignore(numeric_limits<streamsize>::max(), '\n');
solve(meal_cost, tip_percent, tax_percent);
return 0;
}
Day 2 Operators Hackerrank Solution in Java
import java.io.*;
import java.math.*;
import java.security.*;
import java.text.*;
import java.util.*;
import java.util.concurrent.*;
import java.util.regex.*;
public class Solution {
// Complete the solve function below.
static void solve(double meal_cost, int tip_percent, int tax_percent)
{
double tip=meal_cost*tip_percent/100;
double tax=meal_cost*tax_percent/100;
int totalCost=(int)Math.round(meal_cost+tip+tax);
System.out.print(totalCost);
}
private static final Scanner scanner = new Scanner(System.in);
public static void main(String[] args) {
double meal_cost = scanner.nextDouble();
scanner.skip("(\r\n|[\n\r\u2028\u2029\u0085])?");
int tip_percent = scanner.nextInt();
scanner.skip("(\r\n|[\n\r\u2028\u2029\u0085])?");
int tax_percent = scanner.nextInt();
scanner.skip("(\r\n|[\n\r\u2028\u2029\u0085])?");
solve(meal_cost, tip_percent, tax_percent);
scanner.close();
}
}
Operators Hackerrank Output
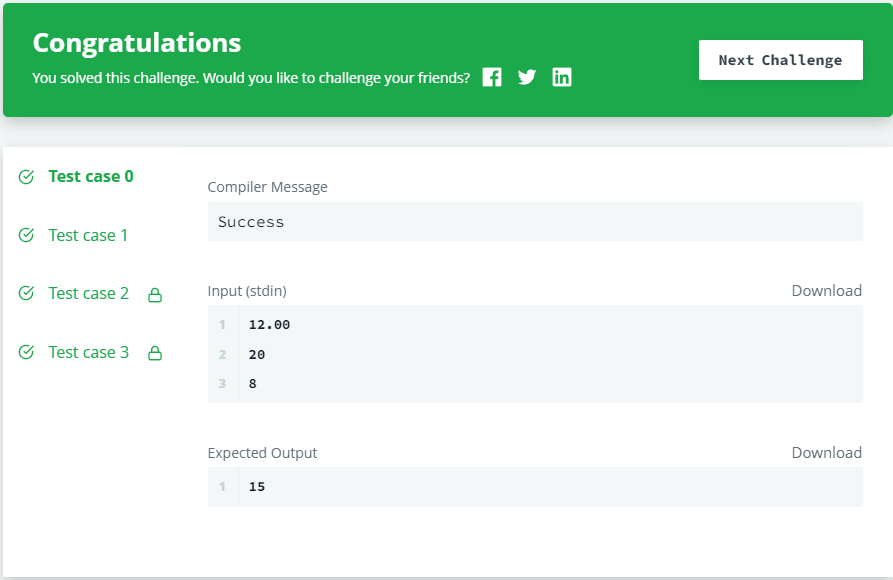
Input Format for Day 2 Operators
There are 3 lines of numeric input:
The first line has a double, mealCost(the cost of the meal before tax and tip).
The second line has an integer, tipPercent(the percentage of mealCost being added as a tip).
The third line has an integer, taxPercent(the percentage of mealCost being added as tax).
Output Format for Operators Hackerrank Solution
Print the total meal cost, where totalCost is the rounded integer result of the entire bill (mealCost with added tax and tip).
Logic
Here we go for day 2 Hackerrank solutions in C. We can solve this problem by taking a simple example. Suppose you are in the cafeteria and you order a meal after you finish your meal you have to pay some amount of money also assume you are also interested to pay a Tip(an extra amount of money almost 15 – 20 per cent of your bill).
You also have to pay taxes so what will be the total amount you paid in the cafeteria? This is a problem for that we will take hacker rank example and solve the problem step by step.
Explanation
As we are taking the hackerrank example Meal cost is 12 dollar (according to hacker rank) Tip per cent is 20 and the tax per cent is 8 so according to the above input our program perform the following steps.
Step:1 Tip = Mealcost * tip percent / 100
Tip = 12 * 20 / 100 = 2.4
Step 2:- Tax = mealcost * tax percent / 100
Tax = 12 * 8 / 100 = 0.96
Step 3:- Total = Mealcost + Tip +Tax.
Total = 12 + 2.4 +0.96 = 15.36
Total = round(Total) = 15.36 So the final Bill is 15 Dollars paid by the customer in the cafeteria. Below is the Day 2 Operators Solution in C Language
More on “30 Days of Code“
Please Like and Share Day 2 Operators Hackerrank Solution of social media.