Write a Simple Anagram Program in Java Using String. We are going to take two strings and compare and check are the 2 strings are anagrams or not. We are also going to know what is an anagram, we are going to solve this program in java using two strings, and for that, we have to compare two strings to check whether they are an anagram or not.
Anagram Program in Java Using String- Table of Content
- What is Anagram
- Anagram Definition
- Example of an Anagrams
- How to Find two Strings are Anagram- Step-by-Step Guide
- Source Code for String Anagram Program in Java
- Java Anagram Program Output
- Anagram Program Explanation Diagram.
What is Anagram
Anagram means to check whether two strings have the same characters or not. In this checking order of the characters does not mandatory to be the same, for example, the first string is “DELL” and the second String is “LLED” both have the same characters so they are Anagram. Below is the Simple Anagram Program in Java Using String in Just 10 Steps.
Anagram Definition
Two Strings Contain the same Characters, Whether Strings order does not need to be same. All characters of one String should be present in the second string to be an Anagram.
–Anagram Definition
Example of an Anagram
- String s1 = “DELL” ;
- String s2 = “LLED”;
These two strings are Anagram. Cause both have the same characters.
- String s3 = “RACE”;
- String s4 = “ARCER”;
These strings are not Anagram. Cause both don’t have the same characters. String3 has 4 characters and String4 has 5 characters.
Simple Anagram Program in Java Step by Step
So As we know now for being a String Anagram number of characters should be the same on both strings. Now we know our first condition. Now we just need to compare the first string characters to the second string, and if all characters meet then Strings are Anagram else Not. If you’re looking to become an expert on Java String, then check out this Java Online Course to get an experience in real-time programming.
Finally, there is a list of 10 steps that may help you to understand the flow of the program, so just followed the Simple Anagram Program in Java Using String in Just 10 Steps.
Step 1: First take one class CkeckAnagramString and import the Arrays package here package is nothing but a group of classes.
Step 2: Then take one main method inside this class we will call the Anagram method by passing two strings in an Anagram method.
Here we can call an Anagram method more than one time by passing another string for checking if any other two strings are an anagram or not. Example Anagram(“Computer”, “Desktop”);
Step 3: This passes a string to store in string1 or string2 variables then the stored string removes all white space by using the replace method.
We can see here that the //s indicates the single whitespace is removed and convert the string into lowercase. After the replacement, the string is stored in the same variable.
Step 4: After that take one variable with any name, this variable type must be Boolean.
Step 5: Take one if statement inside this statement provides one condition. string1 is not equal to string2. Here str.length() we are finding the first string length and comparing the length of the second string.
If both strings are not equal then if the part is executed then the status value becomes false. If both strings are equal then the else part will be executed.
Step 6: Inside the else part, we take two charArray names of this Array Arrays1 and Arrays2. The use of this Array is storing the characters storing we convert this first passing string “keep” into charArray by using the toCharArray method().
Simple Anagram Program in Java Using String; The string character converts into the lower case by using of toLowerCase() method and storing in ArrayS1 after that passing the second string also follows the same approach after that stored in ArrayS2.
Steps 7: After that sort an ArrayS1 and ArrayS2 by using the sort() method.
Step 8: ArrayS1 and ArrayS2 store Characters are compared by using of equals method because of equals method compares the string character-wise if both strings are equal then its return true is stored in the status variable.
For Understand the Flow of the Program you have to test your program multiple times.
Step 9: Hence If both strings contain characters then this status variable stores true after comparing both strings.
Last Step 10: Take one if statement and pass this if statement block status variable, if it is true then it is executed the if part prints the string, are Anagram otherwise else part is executed then the print strings are not an Anagram.
Are you looking for Java help online? Feel free to check this site to have your Java homework done by programming experts.
Code for String Anagram Program in Java
import java.util.Scanner;
public class Anagram
{
public static void main(String[] input)
{
String string1, string2;
int l, length1, length2;
int i, j, count=0, temp=0;
Scanner scan = new Scanner(System.in);
System.out.print("Enter the First String: ");
string1 = scan.nextLine();
System.out.print("Enter the Second String: ");
string2 = scan.nextLine();
length1 = string1.length();
length2 = string2.length();
if(length1 == length2)
{
l = length1;
for(i=0; i<l; i++)
{
count = 0;
for(j=0; j<l; j++)
{
if(string1.charAt(i) == string2.charAt(j))
{
count = 1;
break;
}
}
if(count == 0)
{
temp = 1;
break;
}
}
if(temp == 1)
{
System.out.print("Strings are not Anagram\n");
}
else
{
System.out.print("Strings are Anagram\n");
}
}
else
{
System.out.print("For Anagram: Both Strings Must have the same number of Characters");
}
}
}
Anagram Program Output

Anagram Program Explanation Diagram
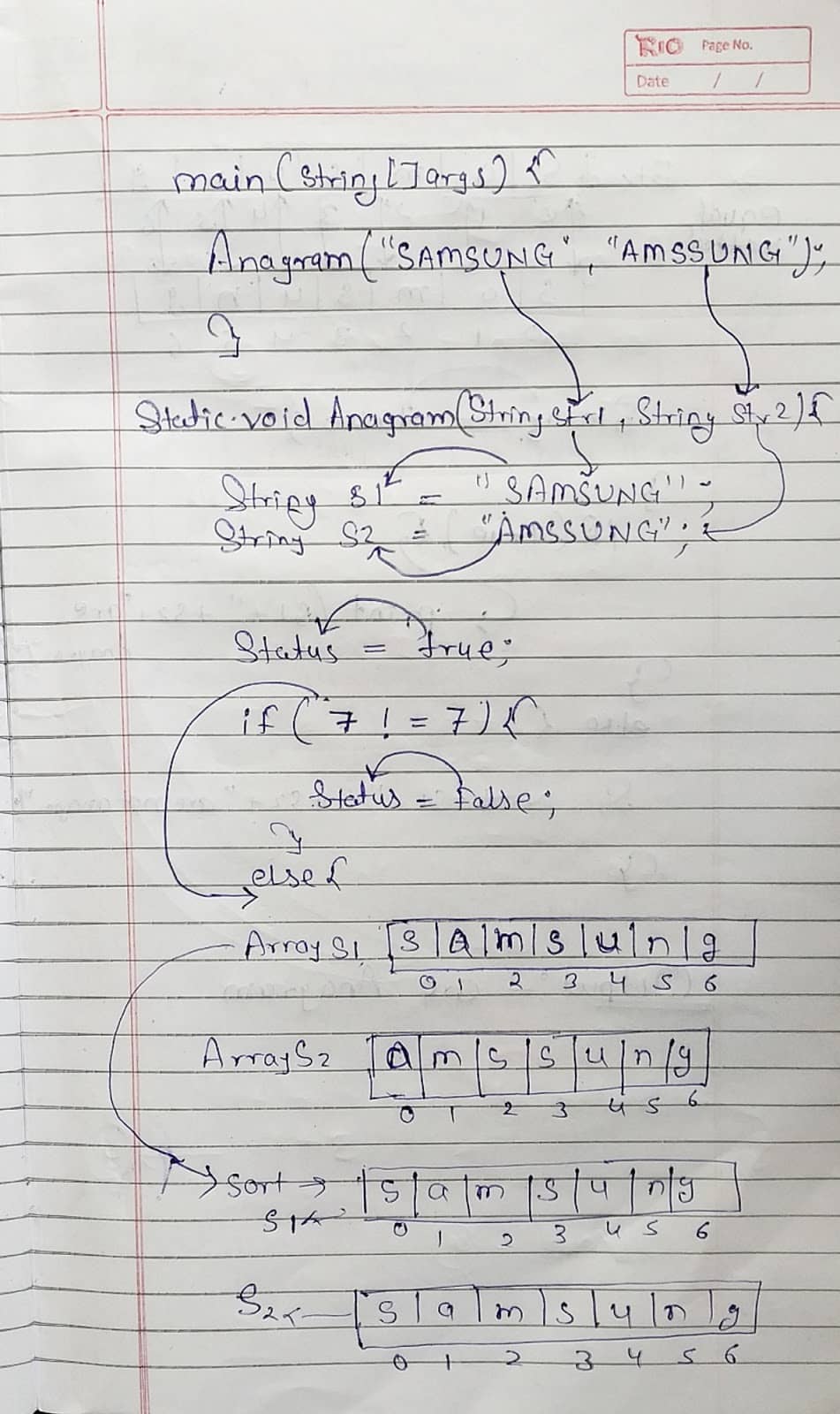
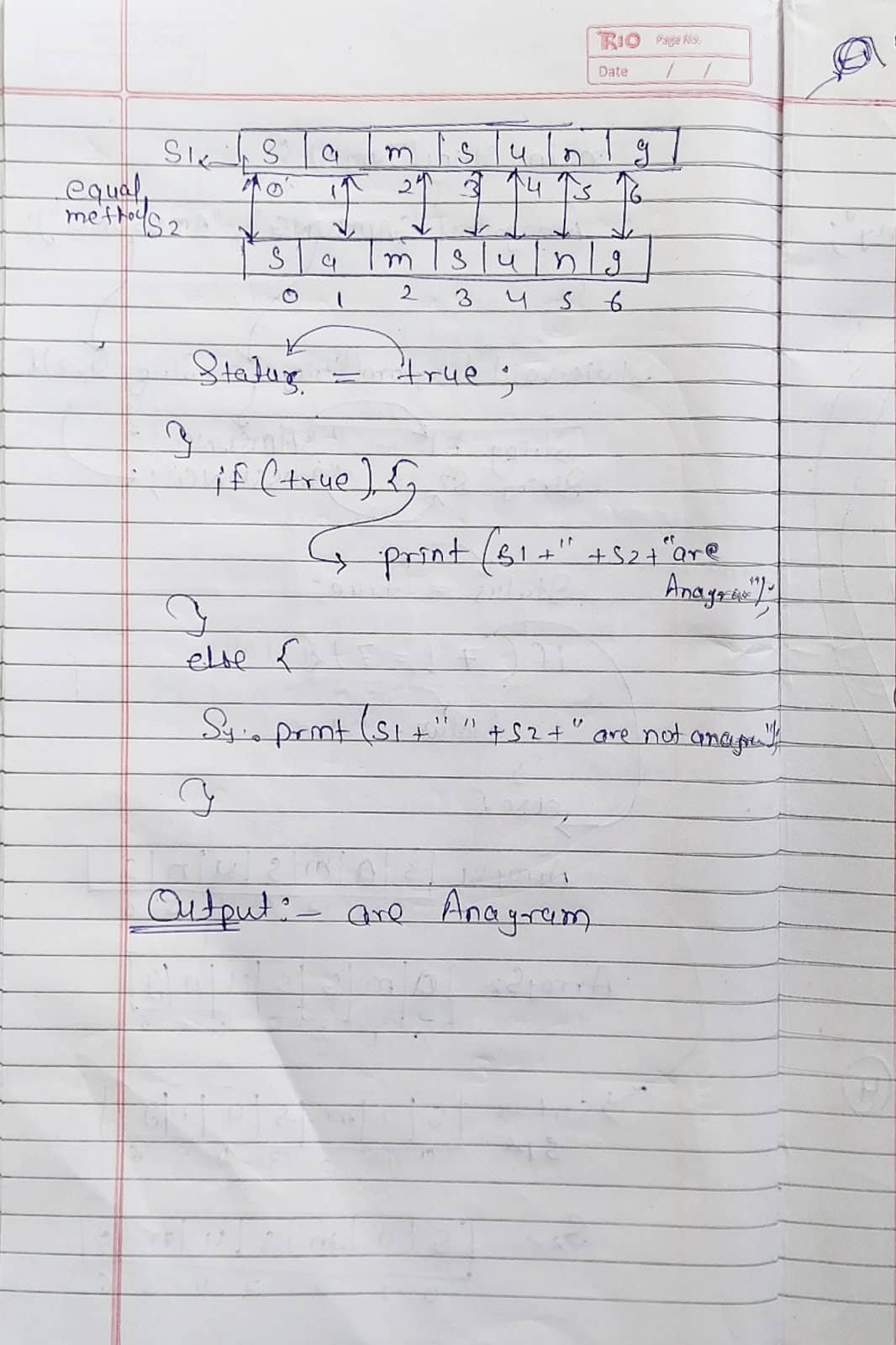
Anagram Test Inputs
Input: 1 String1 = “SAMSUNG” and String2 = “AMSSUNG”
Output: Anagram
Input: 2 String1 = “Shubham” and String2 = “Shubhamtirole”;
Output:- Strings are not Anagram
I hope you like the Simple Anagram Program in Java Using String and the comparison of the program.
Great article found on anagrams, so much detail
Excellent content …super explanation
Keep it up 👍
A Big Thanks to Mr. Pawan We really Appreciate your words, We are working hard to make this platform, Please keep Visit and Learn and Share your Knowledge with Us.