Get the Solution in All three Programming Languages(C, C++. and Java). Day 1 Data Types Hackerrank Solution in C Language. Java Data Types Hackerrank is the Hackerrank second-day problem we have to find the Solution in all three given languages.
Declare 3 variables one of type int, one of type double, and one of type String. Read 3 lines of input from stdin (according to the sequence given in the Input Format section below) and initialize your 3 variables.
Use the + operator to perform the following operations: Print the sum of i plus your int variable on a new line. Print the sum of d plus your double variable to a scale of one decimal place on a new line. Concatenate s with the string you read as input and print the result on a new line.
Table of Contents
Day 1 Data Types Hackerrank Solution Approach
So here is the logic of the problem Hackerrank Solution For 30 Days of Code Day 1 Data Types. Here in this problem, some data type is already defined they are following, int, double, and string and we have to initialize another data type which is the same int, double, and string for the first data type int we have to perform addition with user input data.
And for double also we have to repeat the same process. and for string first, we have to print the already initialize string which is “HackerRank ” and after the “HackerRank” the user string will be shown for this Day 1: Data Types solution I am going to explain the program step by step.
Data Types Hackerrank Problem Explanation
as we know that int i = 4; is already defined so we have to take another integer j, after that we will take user input and store the value in j then we will add the i + j value like below.
int i = 4;
int j = 12;
cout << i + j <<endl;
So the i + j value will be added and print the sum of both numbers.
Now repeat the same process for the double data type so add both double value fixed value and user input value and print the output but remember as you know that the double-precision value is more than 1.
So this is a problem for avoiding this type of problem we can fix the double value by using setprecision(value) means how many digits you want after the pointer and fraction number.
Here you can see that we fixed the value to one that we use setprecision(1).
double d = 4.0;
double e = 4.0;
cout<<fixed<<setprecision(1)<<d<<endl;
Now next step is for the string to be very simple we can print the first string and then the user input sting but we have one problem.
We can not accept space user input string so for that we use getline(cin,t). if you don’t know about the getline() function read Below.
Use of getline in C++
getline is used to store multiple strings with included space and next-line. getline reads line by line and stores the string in the variable and cin is used for storing anything in C++.
Syntax of getline
getline(cin, input_string)
string s = " HackerRank ";
string t ; // user input.
cout<<s<<t;
I hope now you can understand the full problem if you have any queries send an email. You can try the Data Types solution on Hackerank Website.
Day 1 Data Types Hackerrank Solution in C
#include <stdio.h>
#include <string.h>
#include <math.h>
#include <stdlib.h>
int main() {
int i = 4;
double d = 4.0;
char s[] = "HackerRank ";
int i2;
double d2;
char s2[100]; // this is not scalable for input of unknown size
// Read inputs from stdin
scanf("%d", &i2);
scanf("%lf", &d2);
scanf("%*[\n] %[^\n]", s2);
// Print outputs to stdout
printf("%d\n", i + i2);
printf("%.01lf\n", d + d2);
printf("%s%s", s, s2);
return 0;
}
Day 1 Data Types C++ Solution
#include <iostream>
using namespace std;
int main()
{
int i = 4;
double d = 4.0;
string s = "HackerRank ";
// Declare second integer, double, and String variables.
int i2;
double d2;
string s2;
// Read and save an integer, double, and String to your variables.
string tmp;
getline(cin, tmp);
i2 = stoi(tmp);
getline(cin, tmp);
d2 = stod(tmp);
getline(cin, s2);
// Print the sum of both integer variables on a new line.
printf("%i\n", i + i2);
// Print the sum of the double variables on a new line.
printf("%.1f\n", d + d2);
// Concatenate and print the String variables on a new line
// The 's' variable above should be printed first.
cout << s + s2 << endl;
return 0;
}
Java Data Types Hackerrank Solution
import java.util.Scanner;
public class DataTypes
{
public static void main(String[] args) {
int i = 4;
double d = 4.0;
String s = "HackerRank ";
Scanner scan = new Scanner(System.in);
/* Declare second integer, double, and String variables. */
int i2;
double d2;
String s2;
/* Read and save an integer, double, and String to your variables.*/
i2 = scan.nextInt();
d2 = scan.nextDouble();
scan.nextLine();
s2 = scan.nextLine();
/* Print the sum of both integer variables on a new line. */
System.out.println(i + i2);
/* Print the sum of the double variables on a new line. */
System.out.println(d + d2);
/* Concatenate and print the String variables on a new line;
the 's' variable above should be printed first. */
System.out.println(s.concat(s2));
scan.close();
}
}
Day 1 Data Types Hackerrank Solution Output
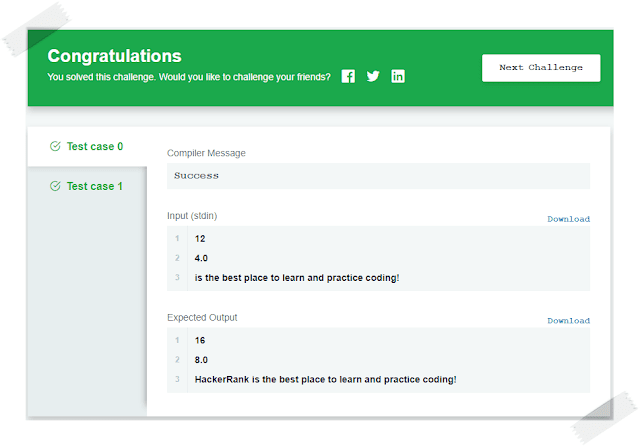
Related to 30 Days of Code
1 thought on “Day 1 Data Types Hackerrank Solution in C C++ & Java | 30 Days of Code”
Comments are closed.